For bugs and new features, use the issue tracker located at GitHub.
Also try the chat room!
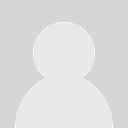
Changing camera type
ddklo wrote at 2011-03-21 19:10:
Hi.
When I change the camera from a projection camera to a orthographic camera by setting the Orthographic property (after Loaded) on the HelixView3D camera movement is no longer possible. Am I missing something or is this a bug?
Regards
Dagfin
ddklo wrote at 2011-04-11 15:10:
Hi.
This issue seemed to be that the Camera property on the CameraController wasn't updated. When I set this manual camera movement works fine. It would be great if this could be the default behavior when changing the Orthographic property, for instance in OnOrthographicChanged in the HelixView3D class.
Regards Dagfinn
objo wrote at 2011-04-12 11:58:
hi Dagfinn, I just changed the CameraController to allow the HelixView3D to change camera. Thanks for the bug report!
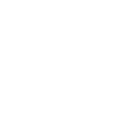
Earth Scale
Hi, I'm trying to create an environment on the scale of earth, i.e. the main object is a map of earth and is 40,000,000 units wide, and I have actual scale buildings only 100 units long. It actually seems to work mostly ok but when I zoom in on the buildings, I get odd clipping effects that appear and disappear while I'm panning or orbiting around the buildings. Any ideas on how best to make this display reliably?
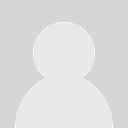
Tearing zoom or rotation of the shape.
DongMini wrote at 2012-08-22 10:02:
Tearing zoom or rotation of the shape.
Why is that?
Is there anyone able to help me?
objo wrote at 2012-08-24 09:47:
Sorry, I don't understand what you mean here. The figure is also missing.
DongMini wrote at 2012-08-24 11:00:
Zoom and Rotation, Capturer the shape of the screen is showing cracks
Left Image
- HelixViewport3D windows screencapturer
Right Image
- Viewprt3DHelper.Export(HelixViewport3D.Viewport, "d:\sample.png", null);
capturer image sample.png
Sample Image url = http://blog.naver.com/web_mini/50148576494
murray_b wrote at 2012-08-28 03:52:
I thought I would add that I get this problem as well.
It happens a lot for me when zooming into a screen of multiple meshes.
I am very interested to see if you can find a solution
objo wrote at 2012-08-28 06:49:
try to reduce the distance between the near and far plane of your camera. This will increase the accuracy of the z-buffer.
http://msdn.microsoft.com/en-us/library/system.windows.media.media3d.projectioncamera.nearplanedistance.aspx
http://msdn.microsoft.com/en-us/library/system.windows.media.media3d.projectioncamera.farplanedistance.aspx
http://en.wikipedia.org/wiki/Z-buffering
http://www.sjbaker.org/steve/omniv/love_your_z_buffer.html
votecoffee wrote at 2014-02-26 02:25:
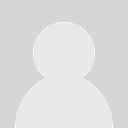
PanoramaCube3D - how to show a skybox
lightxx wrote at 2013-02-26 13:16:
I know that there's a sample included in the source code but I just can't get it to work using my own skybox images. the problem is the cube always looks like - well - a cube, and there are thin black lines where the sides of the cube meet.
Has anyone ever used the PanoramaCube3D for skyboxing and could provide a sample source code? FYI, there are some free skyboxes over here http://www.3delyvisions.com/skf1.htm
balameeta wrote at 2013-03-25 08:54:
The solution is as follows:
In your XAML, add this to your HelixViewPort3D:
RenderOptions.BitmapScalingMode="HighQuality" RenderOptions.EdgeMode="Aliased"
Your code should end up looking something like this: <ht:HelixViewport3D x:Name="viewPort" CameraMode="FixedPosition" RenderOptions.BitmapScalingMode="HighQuality" RenderOptions.EdgeMode="Aliased">
<ModelVisual3D>
<ModelVisual3D.Content>
<AmbientLight Color="White"/>
</ModelVisual3D.Content>
</ModelVisual3D>
<ht:PanoramaCube3D Source="Models\GrandHotel\" >
</ht:PanoramaCube3D>
</ht:HelixViewport3D>
That should fix the problem. The black lines will disappear completely. You MUST have both the BitmapScalingMode and the EdgeMode set for this to work.
All the best.
Amun
lightxx wrote at 2013-03-25 08:58:
balameeta wrote at 2013-03-25 10:06:
All the best for your project!
Amun
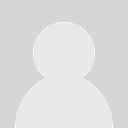
Gradient fill on 3D objects
SeanV12 wrote at 2013-06-06 22:46:
So if anyone can help, how would you give this tube a gradient?
TubeVisual3D tube1 = new TubeVisual3D();
tube1 .Path = new Point3DCollection();
tube1 .Path.Add(new Point3D(0, 0, 0));
tube1 .Path.Add(new Point3D(10, 10, 0));
tube1 .Diameter = 5;
Thanks for reading, and thank you for the toolkit.
objo wrote at 2013-06-07 12:10:
TextureCoordinates
property on the TubeVisual3D
. Set the texture coordinates for each point and use a gradient material!
See the StreamLines demo (but this demo uses the
MeshBuilder
, not the
TubeVisual3D
).SeanV12 wrote at 2013-06-08 16:15:
SeanV12 wrote at 2013-06-18 00:22:
For reference, what I'm trying to do is to make certain points along a tube's path different colors.
objo wrote at 2013-06-18 22:05:
The x-value is set by the specified values (should be normalized 0..1), and the y value corresponds to the position around the tube.
SeanV12 wrote at 2013-06-19 20:37:
Your quick replies are much appreciated, by the way!
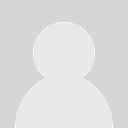
Can you provide 'snapping' for Manipulators ?
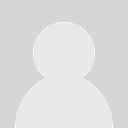
ViewPort printing
mpolitis wrote at 2011-10-16 06:06:
How can i make printings of an Helix viewport, passing the visual tree to the PrintVisual method of the PrintDialogPrintDialog class ? Or with any method you preconize ? Thank's a lot for your impressive toolkit. Michel.
objo wrote at 2011-10-16 20:26:
hi Michel,
yes, printing should be very easy - use the PrintDialog and PrintVisual.
I just tested the following code in ExportDemo:
private void Print_Click(object sender, RoutedEventArgs e) { var dlg = new PrintDialog(); if (dlg.ShowDialog().GetValueOrDefault()) { dlg.PrintVisual(view1.Viewport, this.Title); } }
where view1 is the HelixViewport3D control.
ps. You could also export the Viewport3D to a bitmap at higher resolution and add this image to your print document.
mpolitis wrote at 2011-10-17 05:35:
Wonderfuf Objo ! i have implemented your solution to the AudioDemo sample that i'm transforming to produce Business Intelligence 3D visualization ! I will give you later some feedback with sample outputs ! Kind regards. Michel.
objo wrote at 2011-10-17 10:08:
cool, send some sample outputs, it is interesting to see how the library is being used!
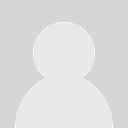
problems when importing 3ds models
jpg99 wrote at 2012-08-15 16:19:
hello,
i use your class StudioReader to import 3ds files. Most of the time the result is very good, but at several occasions i encountered files that were incorrectly imported.
I don't have 3d studio max, i use the freeware 3D Object Converter to test the 3ds files. And i use your sample application, 3D model viewer, to see 'your' version of the file.
I import house models from the site archive3d.net . It's all free and i know that quality is not guaranteed. For example when reading http://archive3d.net/?a=download&id=599a4f07 , 3D Object Converter warns that 40 bad polygons were found. And indeed when viewing the result there are clearly errors
But in some cases the 3ds file is correctly displayed in 3D Object Converter, but not in your 3D model viewer : http://archive3d.net/?a=download&id=e190fe70
objo wrote at 2012-08-24 09:51:
Thanks for the links, I have added the following issue http://helixtoolkit.codeplex.com/workitem/9965
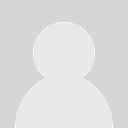
How to draw a pyramid using two points?
mcab21 wrote at 2014-02-04 16:26:
I want to write a method that takes in two parameters, point1 and point2 (XYZ points), and that the method will draw an pyramid. The origin point will be point1 and the end points will be the square with point2 in the centre.
Any ideas how to do this? I see the one AddPyramid method already but it only takes one point.
Thanks in advance!
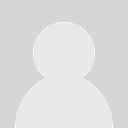
TerrainDemo
icube wrote at 2011-06-21 15:16:
Hi,
Looking at the TerrainDemo project, it uses a .btz file. How can i create such a file? Can't really find anything on google regarding this...
And also, could you do a MultiTouchDemo that uses multi touch input to navigate the HelixView3D.
Thanks for this awesome project.
Regards
objo wrote at 2011-06-22 10:18:
.btz is just a gzip compressed .bt file (binary terrain file). It is only used inside Helix toolkit to reduce the size of the .bt files. You can use GZipStream to compress a .bt file, and the TerrainModel class should be able to read it. You find the TerrainModel class in Visual3Ds/Composite/TerrainVisual3D.cs
Note that this class does not implement a level of detail algorithm, so it is only useful for small models. (You can find LOD implementations on http://www.vterrain.org/)
For documentation on .bt files, see http://www.vterrain.org/Implementation/Formats/BT.html
A multi-touch demo would be very interesting, but I don't have any hardware to test it on myself!
Customer support service by UserEcho