For bugs and new features, use the issue tracker located at GitHub.
Also try the chat room!
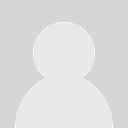
Control Zoom in/out
veerammalkumaran wrote at 2014-08-07 12:11:
IsZoomEnabled option is disabled the zoom in/out, but I want prevent the user from zoom in/out infinitely.
Is there any way to do this...?
everytimer wrote at 2014-08-08 01:31:
veerammalkumaran wrote at 2014-08-08 08:31:
we can disable based on the camera movements, once disabled the camera won't move then when that should be enabled.
veerammalkumaran wrote at 2014-08-08 09:47:
private void viewPort3D_PreviewMouseWheel(object sender, MouseWheelEventArgs e)
{
if (viewPort3D.Camera.LookDirection.Length >= 800 && e.Delta<0)
{
e.Handled = true;
}
if (viewPort3D.Camera.LookDirection.Length <= 15 && e.Delta > 0)
{
e.Handled = true;
}
}

Show wireframe as quads with Helix and SharpDX
Is it possible to get this effect ( Connected Mesh to QuadFaces ) in the viewportDX when a .OBJ model is readed on it?.
I'm using HelixToolkit.Wpf.SharpDX.
Somebody can post a example?
Thanks in advance.
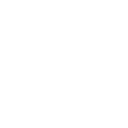
Selidbe - saveti, preselite se što lakše
Put do preseljenja u novi dom prati dosta obaveza, organizacije, rada pa cesto kod vecine ljudi I dosta stresa I napora. Ako se svi potrebni radovi u novom stanu ili kuci zavrse na vreme od useljenja mozete napraviti cak I zabavnu akciju u kojoj ce naravno ucestvovati cela porodica.
Vratimo se na prvobitno stanje. Jos uvek ste u svom trenutnom zivotnom prostoru I predstoji Vam selidba. Najvise bi Vam odgovarao carobni stapic kojim biste se jednim potezom prebacili na novu destinaciju ali… Naoruzajte se voljom za rad I krenite I to prvo od onih stvari I predmeta koji Vam u ovom trenutku nisu potrebni. Kutija po kutija I Vi se polako oprastate od Vase dnevne, spavace sobe I ostalih starih prostorija za koje Vas veze gomila uspomena. Ako svaki spakovani paket, kutiju ili kesu obelezite odnosno napisete sta ste unutra spakovali to ce Vam znatno olaksati raspakivanje.
Nakon toga morate napraviti precizan plan o transportu svih pripremljenih paketa kao i namestaja, tehnike I sl. U eri kompjutera I interneta to je veoma jednostavno, jednim klikom po Vasoj tastaturi pronadjite ekipu koja ce selidbu obaviti u najkracem roku.
Ako ste sve pripremili, radnici firme selidbe beograd koja se na profesionalan nacin bavi svim vrstama selidbi moze biti ispred Vasih vrata. Nakon iznosenja I utovara, kamion sa Vasim stvarima moze da krene. Vama nista drugo ne preostaje vec da svoj dosadasnji dom zakljucate I krenete, I naravno sa sobom ponesete lepe uspomene iz njega.
Niste ni trepnuli a Vi ste vec u svom novom domu I vreme je za raspakovanje. Kako ne bi doslo do nereda I zbrke sada kada ste medju gomilom kutija I paketa najbolje je drzati se sistema koji ste pratili kao I kada ste se pakovali. Ponovo kutija po kutija, bez zurbe I napetosti I nove prostorije ce polako bivati opremljene. Posto niste u mogucnosti odmah da spremate obroke I kuhinju za tako kratko vreme osposobite za koriscenje, porucite nesto od hrane, spakujte decu u krevet I u miru provedite ostatak veceri.

Does Helix Toolkit work on UWP?
Hi everyone,
Just a quick question, does Helix Toolkit work on UWP? I've tried to build it, but lots of errors, so before I start looking into it further, is it even supposed to work?
Helix Toolkit has been amazing on WPF, just want to know if I can use on a UWP app?
Thanks
Garry
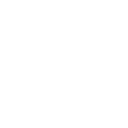
Reference bugs in examples/source?
Hi I just downloaded the newest source of the helix-toolkit. I wanted to get through all the examples to learn about the application, but unfortunately I get a lot of reference errors and I do not know why. I could solve most of them by reinstalling NuGetPackages and adjusting the fakes assemblies, however there are still a lot left. For example in MainViewModel:BaseViewModel class I get the reference error:
Error CS0246 The type or namespace name 'Camera' could not be found (are you missing a using directive or an assembly reference?) DemoCore _NET40 C:\..\helix-toolkit-master\Source\Examples\WPF.SharpDX\DemoCore\BaseViewModel.cs 29 Active.
This goes on with RenderTechnique, IEffectsManager and so on.
Do you have any solution? My references in DemoCore_NET40 all seem to work fine, at least I am getting no yellow symbol in the solution explorer...
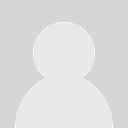
Is there a way to create extruded 3d solid from planar polygon?
luanshixia wrote at 2013-03-02 03:35:
Rossie wrote at 2013-03-04 01:06:
public static List<int> FindBorderEdges(MeshGeometry3D mesh)
{
var dict = new Dictionary<ulong, int>();
for (int i = 0; i < mesh.TriangleIndices.Count / 3; i++)
{
int i0 = i * 3;
for (int j = 0; j < 3; j++)
{
int index0 = mesh.TriangleIndices[i0 + j];
int index1 = mesh.TriangleIndices[i0 + (j + 1) % 3];
int minIndex = Math.Min(index0, index1);
int maxIndex = Math.Max(index1, index0);
ulong key = CreateKey((UInt32)minIndex, (UInt32)maxIndex);
if (dict.ContainsKey(key))
{
dict[key] = dict[key] + 1;
}
else
{
dict.Add(key, 1);
}
}
}
var edges = new List<int>();
foreach (var kvp in dict)
{
// find edges only used by 1 triangle
if (kvp.Value == 1)
{
uint i0, i1;
ReverseKey(kvp.Key, out i0, out i1);
edges.Add((int)i0);
edges.Add((int)i1);
}
}
var borderPoints = new List<Point>();
mesh.Positions.ToList().ForEach(p => borderPoints.Add(new Point(p.X, p.Y)));
var result = OrganizeEdges(edges, borderPoints);
return result;
}
private static List<int> OrganizeEdges(List<int> edges, List<Point> positions)
{
var visited = new Dictionary<int, bool>();
var edgeList = new List<int>();
var resultList = new List<int>();
var nextIndex = -1;
while (resultList.Count < edges.Count)
{
if (nextIndex < 0)
{
for (int i = 0; i < edges.Count; i += 2)
{
if (!visited.ContainsKey(i))
{
nextIndex = edges[i];
break;
}
}
}
for (int i = 0; i < edges.Count; i += 2)
{
if (visited.ContainsKey(i))
continue;
int j = i + 1;
int k = -1;
if (edges[i] == nextIndex)
k = j;
else if (edges[j] == nextIndex)
k = i;
if (k >= 0)
{
var edge = edges[k];
visited[i] = true;
edgeList.Add(nextIndex);
edgeList.Add(edge);
nextIndex = edge;
i = 0;
}
}
// calculate winding order - then add to final result.
var borderPoints = new List<Point>();
edgeList.ForEach(ei => borderPoints.Add(positions[ei]));
var winding = CalculateWindingOrder(borderPoints);
if (winding > 0)
edgeList.Reverse();
resultList.AddRange(edgeList);
edgeList = new List<int>();
nextIndex = -1;
}
return resultList;
}
public static MeshGeometry3D Extrude(MeshGeometry3D surface, double z)
{
var borderIndexes = MeshGeometryHelper2.FindBorderEdges(surface);
var borderPoints = new List<Point3D>();
borderIndexes.ToList().ForEach(bi => borderPoints.Add(surface.Positions[bi]));
var topPoints = borderPoints.ToList();
var botPoints = borderPoints.Select(p => new Point3D(p.X, p.Y, p.Z + z)).ToList();
var allPoints = new List<Point3D>();
var allIndexes = new List<int>();
var allNormals = new List<Vector3D>();
// sides.
allPoints.AddRange(topPoints);
allPoints.AddRange(botPoints);
for (int i = 0; i < topPoints.Count; i +=2)
{
int j = (i + 1) % topPoints.Count;
allIndexes.Add(i);
allIndexes.Add(j);
allIndexes.Add(topPoints.Count + j);
allIndexes.Add(topPoints.Count + j);
allIndexes.Add(topPoints.Count + i);
allIndexes.Add(i);
var a = allPoints[i].ToVector3D();
var b = allPoints[j].ToVector3D();
var c = allPoints[topPoints.Count + j].ToVector3D();
var n0 = b.Subtract(a).Cross(c.Subtract(a)).Unit();
allNormals.Add(n0);
allNormals.Add(n0);
allNormals.Add(n0);
allNormals.Add(n0);
}
var surfaceNormals = new List<Vector3D>();
if (surface.Normals == null)
surface.TriangleIndices.ToList().ForEach(i => surfaceNormals.Add(new Vector3D(0, 0, 1)));
// top
var count = allPoints.Count;
var topSurfacePoints = surface.Positions.Select(p => new Point3D(p.X, p.Y, p.Z + z)).ToList();
var topNormals = surfaceNormals.ToList();
allPoints.AddRange(topSurfacePoints);
allNormals.AddRange(topNormals);
var topSurfaceIndexes = surface.TriangleIndices.Select(i => count + i).ToList();
AddTriangleIndexes(topSurfaceIndexes, allIndexes, false);
// bottom
count = allPoints.Count;
var botSurfacePoints = surface.Positions.ToList();
var botNormals = surfaceNormals.Select(n => n.Flip()).ToList();
allPoints.AddRange(botSurfacePoints);
allNormals.AddRange(botNormals);
var botSurfaceIndexes = surface.TriangleIndices.Select(i => count + i).ToList();
AddTriangleIndexes(botSurfaceIndexes, allIndexes, true);
var mesh = new Mesh3D(allPoints, allIndexes);
var meshGeom = mesh.ToMeshGeometry3D();
meshGeom.Normals = new Vector3DCollection(allNormals);
if (z < 0)
ReverseWinding(meshGeom);
var simple = Simplify(meshGeom);
return simple;
}
private static void AddTriangleIndexes(List<int> triangleIndices, List<int> allIndexes, bool reverseWindingOrder)
{
for (int i = 0; i < triangleIndices.Count; i += 3)
{
var i0 = triangleIndices[i + 0];
var i1 = triangleIndices[i + 1];
var i2 = triangleIndices[i + 2];
if (reverseWindingOrder)
allIndexes.AddRange(new[] { i2, i1, i0 });
else
allIndexes.AddRange(new[] { i0, i1, i2 });
}
}
public static void ReverseWinding(MeshGeometry3D mesh)
{
var indices = mesh.TriangleIndices.ToList();
var flippedIndices = new List<int>();
AddTriangleIndexes(indices, flippedIndices, true);
mesh.TriangleIndices = new System.Windows.Media.Int32Collection(flippedIndices);
}
/// <summary>
/// returns 1 for CW, -1 for CCW, 0 for unknown.
/// </summary>
public static int CalculateWindingOrder(IList<Point> points)
{
// the sign of the 'area' of the polygon is all we are interested in.
var area = CalculateSignedArea(points);
if (area < 0.0)
return 1;
else if (area > 0.0)
return -1;
return 0; // error condition - not even verts to calculate, non-simple poly, etc.
}
public static double CalculateSignedArea(IList<Point> points)
{
double area = 0.0;
for (int i = 0; i < points.Count; i++)
{
int j = (i + 1) % points.Count;
area += points[i].X * points[j].Y;
area -= points[i].Y * points[j].X;
}
area /= 2.0;
return area;
}
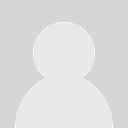
Migrate to HelixToolKit WPF to HelixToolKit SharpDX
cedrelo wrote at 2014-02-07 08:50:
First really thanks for your work!!!!
I use HelixToolKit WPF to develop an WPF application of robot trajectory, i use import of .stl, camera, viewport3D, LineVisual3Ds.
It's works really great, but when we load large data and a lots of stl, the perfomance fall on
some computer.
Do you think it's possible to migrate the projet to the fork HelixToolKit SharpDX to improve perfomance ?
Does the fork has all the functionnality of the orginal project ?
It is possible to use HelixToolKit SharpDX inside a WPF application ?
Thanks
Cedre
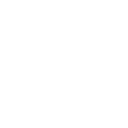
"Cacusjack
"Cacusjack" is a well-known brand in the music and fashion industries. Many people are talking about it, and I'm interested in purchasing their clothes. Can you help me find some great clothing items from this brand?"
Customer support service by UserEcho