Your comments
Yes, its possible.
If you work with a complex material, you need to create a MaterialGroup and Add all materials that will make your final material.
To apply the material in your model, you need to do this (Vb.net)
TryCast(mObj.Content, GeometryModel3D).Material = MaterialGroup
TryCast(mObj.Content, GeometryModel3D).BackMaterial = MaterialGroup
If you don't need back, just does not use them.
Example Basic Material with Image:
Dim b As New ImageBrush(TryCast(myImage, BitmapImage))
The properties Normals, TextureCoord and Positions are private in Helix DLL, but you can change the code and rebuild, or you can create your own class in your code, copying the code from helix.
The helix works with meshs, so, after you importer the .obj, you have a model. So, you don't work no more with the obj file. You can edit and after export again to another .obj.
Other thing thats important remember: If the obj does not have the normals vector, you have to generate them. When you open the .obj in notepad, you can see if haves normals or not.
A mesh is basically defined for positions, triangleIndices and textureCoord. But you can define the vectors normals too, to build a completed mesh. You can define de normals to each position or to each face, thats depends how do you want your model.
I will explain how works MeshBuilder Class:
1) Positions - Collection of vertices
2) TriangleIndices - Collection of indices from de list of positions. Ex: 1 3 2 means that will be build a triangle with the position 1, 3 and 2 from the list of positions, in this order. (The order has influence to decide if the triangle is in back-side or front-side)
3) TextureCoord - Collection of texture coord. The texture coord works with a point (2D - UV coord)
4) Normals - Collection of vectors normals. The vectors normals has big influence in your mesh. I recommend you to study this, like texture coordinates too.
This is the basic to programer 3D.
To zoom Extents:
CameraHelper.ZoomExtents(helix.Camera, helix.Viewport, 1000)
To change Camera:
cameraPerspective.LookDirection = New Vector3D(0, -10, 0)
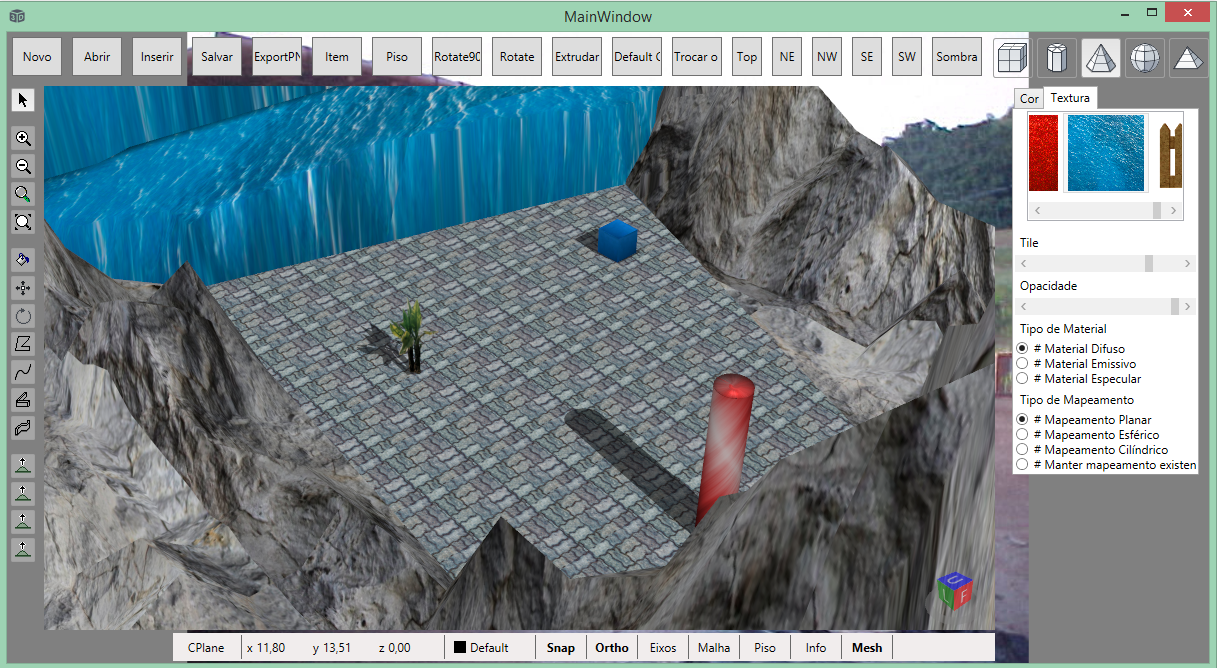
'All borders are a global variable because have the same Material
Dim border As New LinesVisual3D
Public Sub addRectangles()
addRectangleWithBorder(New Point3D(0, 0, 0), 10, 5)
helix.Children.Add(border)
End Sub
Sub addRectangleWithBorder(ByVal position As Point3D, ByVal width As Double, ByVal Lenght As Double)
Dim rectangle As New RectangleVisual3D
rectangle.Length = Lenght
rectangle.Width = width
rectangle.Origin = New Point3D(0, 0, 0)
rectangle.Material = Materials.Red
rectangle.BackMaterial = Materials.Red
Dim p1, p2, p3, p4 As Point3D
p1 = New Point3D(Lenght / 2, width / 2, 0)
p2 = New Point3D(Lenght / 2, -width / 2, 0)
p3 = New Point3D(-Lenght / 2, -width / 2, 0)
p4 = New Point3D(-Lenght / 2, width / 2, 0)
With border
.Thickness = 2
'Base
.Points.Add(p1)
.Points.Add(p2)
.Points.Add(p2)
.Points.Add(p3)
.Points.Add(p3)
.Points.Add(p4)
.Points.Add(p1)
.Points.Add(p4)
.Color = Colors.Black
End With
helix.Children.Add(rectangle)
End Sub
Customer support service by UserEcho
Put this in your component .MouseDown Event:
Dim o as Object = helixViewport.FindNearestVisual(e.GetPosition(helixViewport))