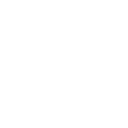
0
Rectangular box with different picture on each face
I'm trying to create a rectangular box visual with a different picture on each face, representing a piece moving around a board.
I currently use a 2D rectangle, but I'd like it to have some width so it doesn't disappear at the wrong angle. I've tried using two rectangles with some filler, but the pieces separate oddly when the movement animation begins.
The front and back faces would be the same (a piece portrait) and the filler is unimportant. The picture can widely vary, as the size of the piece is scaled to the picture's ratio.
What visual should I use? How would I set this up?
I currently use a 2D rectangle, but I'd like it to have some width so it doesn't disappear at the wrong angle. I've tried using two rectangles with some filler, but the pieces separate oddly when the movement animation begins.
The front and back faces would be the same (a piece portrait) and the filler is unimportant. The picture can widely vary, as the size of the piece is scaled to the picture's ratio.
What visual should I use? How would I set this up?
Customer support service by UserEcho
I ended up extending BoxVisual3D. The texture coordinates, in the case of this particular Visual3D, each refer to a face of the cube. Those faces who receive an 'empty' set of 0,0 TextureCoordinates are uniform in color, based on the source image. I found this non-distracting enough to not try and solve.
The front and back face, in my example, receive a specific ordered set of texture points that cause the image to be displayed in the proper upright fashion for the user.
Note that creating a new point collection each time is not a good approach, as Tessellate() is called constantly.
The code is below.
class FaceBox : BoxVisual3D