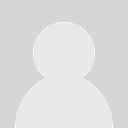
0
some bugs found in MeshBuilder
This discussion was imported from CodePlex
jpg99 wrote at 2012-08-15 15:17:
hello,
i'm using your toolkit for a project at work . I have to draw 3d houses from a 2D polygon (plane section of the house), so i experimented with AddExtrudedGeometry, and encountered several difficulties. Eventually i wrote my own function for building the 3d houses, and i don't remember precisely what the problems were (sorry it was a few weeks ago), mostly problems with triangles defined in the wrong sense so that the outside / inside were inversed.
Here is the code i corrected in MeshBuilder.cs :
public void AddExtrudedGeometry(IList points, Vector3D xaxis, Point3D p0, Point3D p1) { var ydirection = Vector3D.CrossProduct(xaxis, p0 - p1); ydirection.Normalize(); xaxis.Normalize(); int np = 2 * points.Count; foreach (var p in points) { int i0 = this.positions.Count; var v = (xaxis * p.X) + (ydirection * p.Y); this.positions.Add(p0 + v); this.positions.Add(p1 + v); v.Normalize(); if (this.normals != null) { this.normals.Add(v); this.normals.Add(v); } if (this.textureCoordinates != null) { this.textureCoordinates.Add(new Point(0, 0)); this.textureCoordinates.Add(new Point(1, 0)); } int i1 = i0 + 1; int i2 = (i0 + 2) % np; int i3 = i2 + 1; this.triangleIndices.Add(i0); this.triangleIndices.Add(i2); this.triangleIndices.Add(i1); this.triangleIndices.Add(i2); this.triangleIndices.Add(i3); this.triangleIndices.Add(i1); } }public void AddTriangleFan( IList fanPositions, IList fanNormals = null, IList fanTextureCoordinates = null) { if (this.positions == null) { throw new ArgumentNullException("fanPositions"); } if (fanNormals != null && this.normals == null) { throw new ArgumentNullException("fanNormals"); } if (fanTextureCoordinates != null && this.textureCoordinates == null) { throw new ArgumentNullException("fanTextureCoordinates"); } int index0 = this.positions.Count; foreach (var p in fanPositions) { this.positions.Add(p); } if (this.textureCoordinates != null && fanTextureCoordinates != null) { foreach (var tc in fanTextureCoordinates) { this.textureCoordinates.Add(tc); } } if (this.normals != null && fanNormals != null) { foreach (var n in fanNormals) { this.normals.Add(n); } } int indexEnd = this.positions.Count; for (int i = index0; i + 2 < indexEnd; i += 3) { this.triangleIndices.Add(i); this.triangleIndices.Add(i + 1); this.triangleIndices.Add(i + 2); } }
objo wrote at 2012-08-24 09:49:
Thanks for the code! I added http://helixtoolkit.codeplex.com/workitem/9964
Customer support service by UserEcho