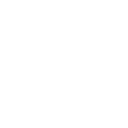
0
Automatically move manipulator
I have created a small example with a "robot" containing rotating axes.
With the three manipulators I can move the axis of the robot.
But I'm looking for a way to move the manipulators when the robot moves.
So when axis 1 of the robot moves you see all the 'stacked' objects moving but not the manipulators.
How can I achieve this?
Here is my source code:
SphereVisual3D sphereAxis1 = new SphereVisual3D();
sphereAxis1.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis1.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
sphereAxis1.Radius = 1.0;
hvpMain.Children.Add(sphereAxis1);
RotateManipulator manipulatorAxis1 = new RotateManipulator();
manipulatorAxis1.Color = Colors.Blue;
manipulatorAxis1.Bind(sphereAxis1);
manipulatorAxis1.Axis = new System.Windows.Media.Media3D.Vector3D(0, 0, 1);
manipulatorAxis1.Diameter = 3.5;
manipulatorAxis1.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis1);
PipeVisual3D pipe1 = new PipeVisual3D();
pipe1.Fill = new SolidColorBrush(Colors.Orange);
pipe1.Diameter = 5.0;
pipe1.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, -1);
pipe1.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
sphereAxis1.Children.Add(pipe1);
PipeVisual3D pipe2 = new PipeVisual3D();
pipe2.Fill = new SolidColorBrush(Colors.Orange);
pipe2.Diameter = 2.0;
pipe2.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
pipe2.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
pipe1.Children.Add(pipe2);
SphereVisual3D sphereAxis2 = new SphereVisual3D();
sphereAxis2.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis2.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
sphereAxis2.Radius = 1.0;
pipe2.Children.Add(sphereAxis2);
RotateManipulator manipulatorAxis2 = new RotateManipulator();
manipulatorAxis2.Pivot = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
manipulatorAxis2.Axis = new System.Windows.Media.Media3D.Vector3D(0, 1, 0);
manipulatorAxis2.Color = Colors.Green;
manipulatorAxis2.Bind(sphereAxis2);
manipulatorAxis2.Position = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
manipulatorAxis2.Diameter = 3.5;
manipulatorAxis2.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis2);
PipeVisual3D pipe3 = new PipeVisual3D();
pipe3.Fill = new SolidColorBrush(Colors.Orange);
pipe3.Diameter = 2.0;
pipe3.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
pipe3.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
sphereAxis2.Children.Add(pipe3);
SphereVisual3D sphereAxis3 = new SphereVisual3D();
sphereAxis3.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis3.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
sphereAxis3.Radius = 1.0;
pipe3.Children.Add(sphereAxis3);
RotateManipulator manipulatorAxis3 = new RotateManipulator();
manipulatorAxis3.Pivot = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
manipulatorAxis3.Axis = new System.Windows.Media.Media3D.Vector3D(0, 1, 0);
manipulatorAxis3.Color = Colors.Green;
manipulatorAxis3.Bind(sphereAxis3);
manipulatorAxis3.Position = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
manipulatorAxis3.Diameter = 3.5;
manipulatorAxis3.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis3);
PipeVisual3D pipe4 = new PipeVisual3D();
pipe4.Fill = new SolidColorBrush(Colors.Orange);
pipe4.Diameter = 2.0;
pipe4.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
pipe4.Point2 = new System.Windows.Media.Media3D.Point3D(7, 0, 7);
sphereAxis3.Children.Add(pipe4);
Kind regards,
Arjan
With the three manipulators I can move the axis of the robot.
But I'm looking for a way to move the manipulators when the robot moves.
So when axis 1 of the robot moves you see all the 'stacked' objects moving but not the manipulators.
How can I achieve this?
Here is my source code:
SphereVisual3D sphereAxis1 = new SphereVisual3D();
sphereAxis1.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis1.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
sphereAxis1.Radius = 1.0;
hvpMain.Children.Add(sphereAxis1);
RotateManipulator manipulatorAxis1 = new RotateManipulator();
manipulatorAxis1.Color = Colors.Blue;
manipulatorAxis1.Bind(sphereAxis1);
manipulatorAxis1.Axis = new System.Windows.Media.Media3D.Vector3D(0, 0, 1);
manipulatorAxis1.Diameter = 3.5;
manipulatorAxis1.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis1);
PipeVisual3D pipe1 = new PipeVisual3D();
pipe1.Fill = new SolidColorBrush(Colors.Orange);
pipe1.Diameter = 5.0;
pipe1.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, -1);
pipe1.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
sphereAxis1.Children.Add(pipe1);
PipeVisual3D pipe2 = new PipeVisual3D();
pipe2.Fill = new SolidColorBrush(Colors.Orange);
pipe2.Diameter = 2.0;
pipe2.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 0);
pipe2.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
pipe1.Children.Add(pipe2);
SphereVisual3D sphereAxis2 = new SphereVisual3D();
sphereAxis2.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis2.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
sphereAxis2.Radius = 1.0;
pipe2.Children.Add(sphereAxis2);
RotateManipulator manipulatorAxis2 = new RotateManipulator();
manipulatorAxis2.Pivot = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
manipulatorAxis2.Axis = new System.Windows.Media.Media3D.Vector3D(0, 1, 0);
manipulatorAxis2.Color = Colors.Green;
manipulatorAxis2.Bind(sphereAxis2);
manipulatorAxis2.Position = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
manipulatorAxis2.Diameter = 3.5;
manipulatorAxis2.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis2);
PipeVisual3D pipe3 = new PipeVisual3D();
pipe3.Fill = new SolidColorBrush(Colors.Orange);
pipe3.Diameter = 2.0;
pipe3.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 2);
pipe3.Point2 = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
sphereAxis2.Children.Add(pipe3);
SphereVisual3D sphereAxis3 = new SphereVisual3D();
sphereAxis3.Fill = new SolidColorBrush(Colors.Blue);
sphereAxis3.Center = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
sphereAxis3.Radius = 1.0;
pipe3.Children.Add(sphereAxis3);
RotateManipulator manipulatorAxis3 = new RotateManipulator();
manipulatorAxis3.Pivot = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
manipulatorAxis3.Axis = new System.Windows.Media.Media3D.Vector3D(0, 1, 0);
manipulatorAxis3.Color = Colors.Green;
manipulatorAxis3.Bind(sphereAxis3);
manipulatorAxis3.Position = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
manipulatorAxis3.Diameter = 3.5;
manipulatorAxis3.InnerDiameter = 2.5;
hvpMain.Children.Add(manipulatorAxis3);
PipeVisual3D pipe4 = new PipeVisual3D();
pipe4.Fill = new SolidColorBrush(Colors.Orange);
pipe4.Diameter = 2.0;
pipe4.Point1 = new System.Windows.Media.Media3D.Point3D(0, 0, 7);
pipe4.Point2 = new System.Windows.Media.Media3D.Point3D(7, 0, 7);
sphereAxis3.Children.Add(pipe4);
Kind regards,
Arjan
Customer support service by UserEcho