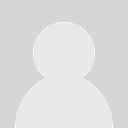
Need Scaling Help
matthewfrazier wrote at 2010-10-12 17:23:
I'm trying to create a simple tube which I can do fine using this wonderful toolkit. What I'm having a problem with is perspective or scaling. Using the given array list of xyz coordinates I draw my tube BUT I can't make it "look right" in my view. I tried dividing by 1000 but that obviously just keeps the same problem just on a smaller scale. My issue is that my z coordinate is always going to be much larger than my xy. I want to create a tubing path for my users to view so they can see the deviation of the tubing. I can do this with a simple xyz scatter plot in winforms but I want to be able to have the 3D camera control in WPF to give the users more perspective. Any ideas?
private void LoadArrayList() { _x = new ArrayList() { 0, 1.31, 2.71, 3.06, 3.48, 4.69, 6.8, 9.51, 12.37, 15.75, 19.65, 23.76, 30.21, 38.89, 47.77, 56.81, 69.86, 78.23, 86.62, 96.26, 107.4, 117.4, 125.63, 133.91, 142.02, 148.47, 152.32 }; _y = new ArrayList() { 0, 4.76, 7.19, 6.56, 6.76, 8.31, 11.66, 16.48, 22.3, 29.07, 36.08, 43.1, 54.16, 69.78, 85.66, 101.81, 124.95, 140.06, 154.96, 169.97, 185.11, 198.52, 210.58, 222.8, 235.48, 245.87, 251.77 }; _z = new ArrayList() { 0, -463.96, -775.94, -901.94, -964.94, -1027.91, -1091.78, -1155.54, -1217.2, -1279.74, -1342.23, -1404.7, -1497.83, -1622.55, -1747.23, -1872.88, -2060, -2184.81, -2309.64, -2434.37, -2558.96, -2683.84, -2808.99, -2934.12, -3059.22, -3161.49, -3221.08 }; } private void BuildTube() { var pc = new Point3DCollection(); for (int i = 0; i < (_x.Count); i++) { pc.Add(new Point3D((Convert.ToDouble(_x[i]) / 1000.00) * 5, (Convert.ToDouble(_y[i]) / 1000.00) * 5, (Convert.ToDouble(_z[i]) / 1000.00) * 5)); } tube.Path = pc; }
objo wrote at 2010-10-13 08:09:
can you change only the scale of the z-coordinates?
var _x = new List<double> { 0, 1.31, 2.71, 3.06, 3.48, 4.69, 6.8, 9.51, 12.37, 15.75, 19.65, 23.76, 30.21, 38.89, 47.77, 56.81, 69.86, 78.23, 86.62, 96.26, 107.4, 117.4, 125.63, 133.91, 142.02, 148.47, 152.32 }; var _y = new List<double> { 0, 4.76, 7.19, 6.56, 6.76, 8.31, 11.66, 16.48, 22.3, 29.07, 36.08, 43.1, 54.16, 69.78, 85.66, 101.81, 124.95, 140.06, 154.96, 169.97, 185.11, 198.52, 210.58, 222.8, 235.48, 245.87, 251.77 }; var _z = new List<double> { 0, -463.96, -775.94, -901.94, -964.94, -1027.91, -1091.78, -1155.54, -1217.2, -1279.74, -1342.23, -1404.7, -1497.83, -1622.55, -1747.23, -1872.88, -2060, -2184.81, -2309.64, -2434.37, -2558.96, -2683.84, -2808.99, -2934.12, -3059.22, -3161.49, -3221.08 }; var pc = new Point3DCollection(); for (int i = 0; i < (_x.Count); i++) { pc.Add(new Point3D(_x[i], _y[i], _z[i]*0.01)); } tube.IsPathClosed = false; tube.Path = pc;
Customer support service by UserEcho