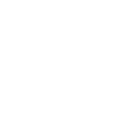
view panning and the coordinate axis symbol
I have a HelixViewport3D window in a WPF app, with Pan and axis coordinate features enabled. I am rendering a 'point-cloud' using PointsVisual3D. When I Pan the point-cloud around, the coordinate axis stays in the center of the view instead of moving with the point cloud, and this seems wrong. I thought that the 'Pan' operation simply translated the camera around in the current viewing plane, which would make everything in the model (including the coordinate axis symbol) move together, but clearly this is not the case.
This screenshot shows the situation: The left-hand HelixViewport3D viewport shows a point collection panned off center, while the right-hand viewport shows the original, unpanned setup.
What am I missing, and what do I need to do to make sure the coordinate symbol stays in the same relative position with respect to other model elements when panning the view?
TIA,
Frank
Customer support service by UserEcho
Add a coordinate system urself, do not use the one of the helixviewport with center/center.
The one of the helixviewport is the global axis and always stays relative to the whole viewport.
Easiest way: in your xaml:
Got it - Thanks! Is there any way at present to add labels to the 'geometry-centered' coordinate system? I saw there was an issue (Issue #18) about this, with the notation that labels were added, but I don't see that anywhere in the available properties for CoordinateSystemVisual3D, nor did I see anything in the class code source. Am I missing something?
Frank
Remember, in no way the coordinate system is geometry centered. It is just a coordinate system positioned at (0,0,0).
No, it's not implemented as far as I know.
What you could do is this:
Or simply write your own CoordincateSystemVisual-class. If you subclass the existing one it shouldn't be that hard.
Thanks for the info. As an exercise, I attempted to write the subclass you suggested, but got stuck on setting the default property for the axis label. A snippet showing the problem is included below:
The above code compiles fine, but the line
shows the error "Default value type does not match tpe of property 'XAxisLabel' :-(
I confess that I really don't know what I'm doing in this DependencyPropery declaration, but I tried to copy from the CoordinateSystemVisual3D declaration as much as possible. What I'm trying to do here is set the default property of the X axis label to "X" - what's the proper way to do this?
Full code for the 'LabeledCoordSysVis3D' class is provided below:
"x" is string
XAxisLabel has type Color... why?
XAxisLabelProperty is registered with type BillboardTextItem.. why?
Egon,
Thanks! With the clues you provided, I have been able to get the subclass working properly. Here's a screenshot of my magnetometer calibration tool, with the left-hand 'raw' viewport coordinate axis symbol created using '<local:LabeledCoordSysVis3D />', and the right-hand 'calibrated' viewport symbol created using
The only visible difference between the two is that the 'X', 'Y', & 'Z' labels on the left are colored the same as the axis arrows, while the ones on the right are all black text.
The next screenshot shows the XAML for both windows and the exposed properties for both the LabeledCoordSysVis3D and CoordinateSystemVisual3D classes
And here is the complete - now working thanks to Egon! - subclass code:
I'm sure others can do a better job than this, but I learned a LOT getting this far, and I really appreciate the help from Egon and others - THANKS!!
Frank
If you don't like the Labels having the same colors as the axis, remove the lines
also maybe think about using zlabel.FontSize instead of zlabel.HeightFactor. I like the result more ;)