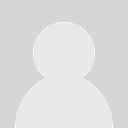
0
Under review
Fill polygon with texture
This discussion was imported from CodePlex
Nikodem wrote at 2014-06-25 22:55:
How can I make 2D polygon geometry with image texture.
For the rectangle I use two triangle and for texture I added coordinates for triangles:
// triangle ABC
mesh.TriangleIndices.Add(0);
mesh.TriangleIndices.Add(1);
mesh.TriangleIndices.Add(2);
// triangle ACD
mesh.TriangleIndices.Add(0);
mesh.TriangleIndices.Add(2);
mesh.TriangleIndices.Add(3);
mesh.TextureCoordinates.Add(new Point(0, 1)); // point A
mesh.TextureCoordinates.Add(new Point(1, 1)); // point B
mesh.TextureCoordinates.Add(new Point(1, 0)); // point C
mesh.TextureCoordinates.Add(new Point(0, 0)); // point D
var d = new DiffuseMaterial(imgBrush);
mGeometries.Add(new GeometryModel3D(mesh, d));
....
On this discussion I found functions for create of polygon.
public static MeshGeometry3D FillPolygon(Polygon p)
Texture is rectangle image and I wants show shape cut of texture on the polygon.
Thank you very much.
Jindrich
For the rectangle I use two triangle and for texture I added coordinates for triangles:
// triangle ABC
mesh.TriangleIndices.Add(0);
mesh.TriangleIndices.Add(1);
mesh.TriangleIndices.Add(2);
// triangle ACD
mesh.TriangleIndices.Add(0);
mesh.TriangleIndices.Add(2);
mesh.TriangleIndices.Add(3);
mesh.TextureCoordinates.Add(new Point(0, 1)); // point A
mesh.TextureCoordinates.Add(new Point(1, 1)); // point B
mesh.TextureCoordinates.Add(new Point(1, 0)); // point C
mesh.TextureCoordinates.Add(new Point(0, 0)); // point D
var d = new DiffuseMaterial(imgBrush);
mGeometries.Add(new GeometryModel3D(mesh, d));
....
On this discussion I found functions for create of polygon.
public static MeshGeometry3D FillPolygon(Polygon p)
{
List<Point3D> pts3D = new List<Point3D>();
foreach (var point in p.Points)
{
pts3D.Add(new Point3D(point.X, point.Y, 0));
}
Polygon3D p3 = new Polygon3D(pts3D);
return FillPolygon(p3);
}
public static MeshGeometry3D FillPolygon(Polygon3D p3)
{
var meshBuilder = new MeshBuilder(false, false);
Polygon polygon = p3.Flatten();
var triangleIndexes = CuttingEarsTriangulator.Triangulate(polygon.Points);
meshBuilder.Append(p3.Points, triangleIndexes);
return meshBuilder.ToMesh();
}
Polygon drawing right, but I do not know how I do configuration for fill texture on the polygon.
Texture is rectangle image and I wants show shape cut of texture on the polygon.
Thank you very much.
Jindrich

0
Michael Powell 11 years ago
I don't know if I am finding a similar issue. Sometimes the material I set it up with appears correctly. Sometimes not; sort of a default pale blue false image, if you will...

0
Under review
Øystein Bjørke 10 years ago
I guess the triangulator is not supporting texture coordinates. That's a new feature that could be added, I think.
Customer support service by UserEcho