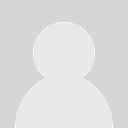
+1
Zoom out
This discussion was imported from CodePlex
Cygor6 wrote at 2012-11-23 22:40:
I'm beginners and would need your help! I made a console application
to save down a rendered image. BUT the camera is too close and
I can not somehow zoom out.
How do I solve this?
using System.IO; using System.Threading; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Shapes; using HelixToolkit.Wpf; namespace ConsoleApplication1 { internal class Program { public static IHelixViewport3D HelixView { get; set; } public static Model3D MyModel { get; set; } private static void Main(string[] args) { var thread = new Thread(Test); thread.SetApartmentState(ApartmentState.STA); thread.Start(); thread.Join(); } private static void Test() { var c = new FileStream("Ferarri40.3ds", FileMode.Open, FileAccess.Read, FileShare.Read); var reader = new StudioReader {TexturePath = "."}; MyModel = reader.Read(c); var m = new ModelVisual3D {Content = MyModel}; const string Path = @"H:\test.png"; const int Height = 480; const int Width = 640; var vp = new HelixViewport3D {Width = Width, Height = Height}; vp.Viewport.Children.Add(m); vp.Children.Add(new DefaultLights()); var zoom = new ScaleTransform3D(1.5, 1.5, 1.5); var t = new Transform3DGroup(); t.Children.Add(zoom); vp.Camera.Transform = t; vp.Camera = CameraHelper.CreateDefaultCamera(); vp.Camera.Position = new Point3D(5.300, -12.300, 3.300); vp.Camera.LookDirection = new Vector3D(-6.300, 11.000, -6.600); vp.Camera.UpDirection = new Vector3D(0.000, 0.000, 1.000); vp.Camera.NearPlaneDistance = 0.123; var r = new Rectangle {Width = vp.ActualWidth, Height = vp.ActualHeight}; var cb = new BitmapCacheBrush(vp) {BitmapCache = new BitmapCache(0)}; r.Fill = cb; r.Arrange(new Rect(0, 0, r.Width, r.Height)); r.UpdateLayout(); vp.Export(Path); } } }
Customer support service by UserEcho